Firebase Cloud Messaging (FCM) allows you to set up push notifications seamlessly, even when your Next.js application is running in the background or entirely closed. Let’s dive in!
1. Initiating FCM
- Navigate to the Firebase Console and create a project.
- Under
Project Settings > General > Firebase SDK snippet > config
, you’ll find the config details. Extract themessagingSenderId
and keep it handy.
2. Incorporate the Firebase SDK
Install the Firebase package for your Next.js application:
yarn add firebase
# or
npm install firebase
3. Establish Connection with Firebase Cloud Messaging
Now, create a webPush.js
in your project directory. This is where you’ll initialize and retrieve the FCM token. We’ll be using localforage
for storing the FCM token:
yarn add localforage
# or
npm install localforage
LocalForage enhances the offline capabilities of web apps by utilizing asynchronous storage.
Your webPush.js
will look like this:
import 'firebase/messaging';
import firebase from 'firebase/app';
import localforage from 'localforage';
const firebaseCloudMessaging = {
// ... (rest of the content)
};
export { firebaseCloudMessaging };
4. Integrating Service Workers
Service workers are essentially intermediaries that can manage HTTP requests from your browser to external content. They’re crucial for push notifications, intercepting them and deciding how to display them to users.
For Next.js apps, a custom server is typically needed to correctly scope the service worker.
To cache the FCM initialization, create public/firebase-messaging-sw.js
:
importScripts('https://www.gstatic.com/firebasejs/7.9.1/firebase-app.js');
importScripts('https://www.gstatic.com/firebasejs/7.9.1/firebase-messaging.js');
// ... (rest of the content)
5. Crafting a Custom Server
To ensure the service worker functions as intended, especially in Next.js, you need to set up a custom server:
const express = require('express');
const next = require('next');
// ... (rest of the server setup)
6. Initializing and Displaying Notifications
Having set up the service worker, you’ll now need to integrate the Firebase configuration to receive notifications. Implement this in your primary app component, utilizing the webPush.js
functions.
7. Testing with POSTMAN
Firebase allows you to push messages directly from your server to your application. This can be easily tested with POSTMAN:
- Grab the FCM server token from
Firebase console > Project Settings > Cloud Messaging
. - In POSTMAN, set up a new POST request to ’https://fcm.googleapis.com/fcm/send’.
- Replace placeholders with the appropriate FCM server and client tokens.
- Hit send, and voilà!
Notifications will appear in your browser’s developer console when your app is active. If it’s running in the background, a brief pop-up notification will appear.
That’s it! Now you’ve successfully integrated Firebase Push Notifications in your Next.js application. Happy coding!
Subscribe now!
Get latest news in regards to tech.
We wont spam you. Promise.
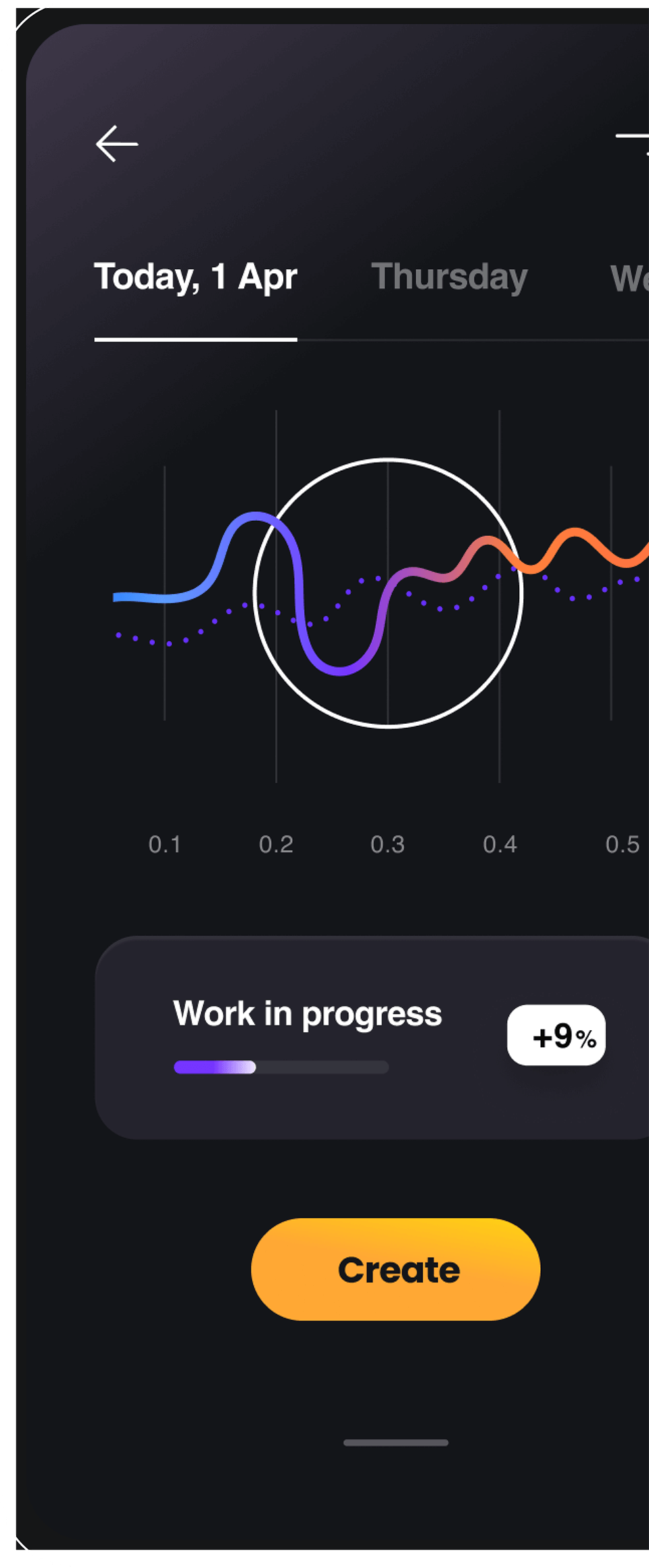
© 2023 Otherside Limited. All rights reserved.